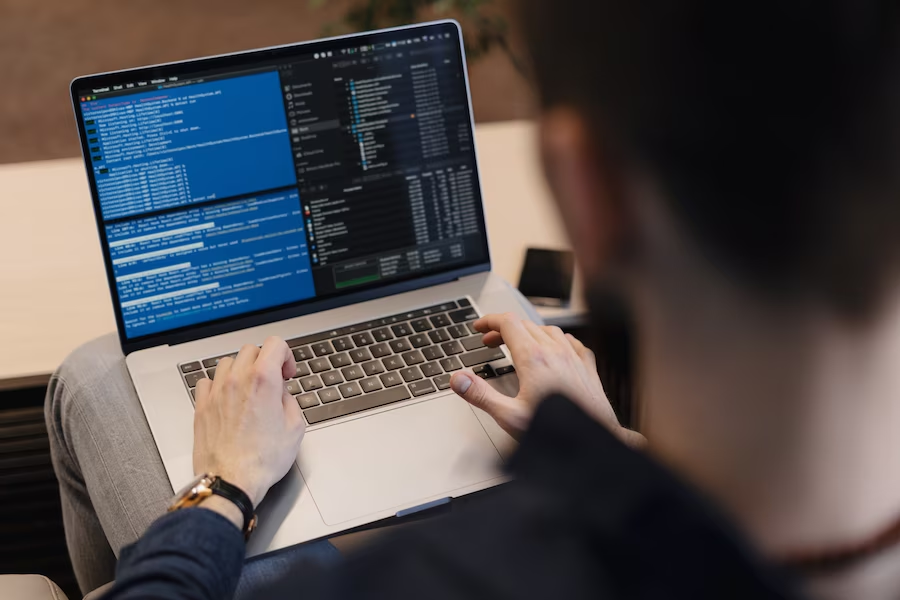
Adding a Splash of Color: A Guide to Manipulating Images in JavaScript
JavaScript, the dynamic and versatile language, has transformed the way we interact with web pages. Among its many capabilities, manipulating images stands out as a particularly engaging feature. In this article, we delve into the fascinating world of handling images in JavaScript. We’ll cover the basics of the image object in JavaScript, explore js images, and learn how to change image src in JavaScript.
Image Object in JavaScript: A Canvas for Creativity
An image object in JavaScript serves as a powerful tool for web developers, transcending mere graphical representation to become a dynamic canvas for creativity. Understanding the intricacies of this object is pivotal for anyone seeking to infuse their web projects with visual allure and functionality.
Creating an Image Object
To initialize an image object in JavaScript, the new Image() constructor is employed. This method instantiates a new HTMLImageElement instance, providing developers with a versatile entity that can be manipulated using an array of JavaScript properties and methods. Below is an example of how to create an image object:
var myImage = new Image(); myImage.src = ‘path/to/your/image.jpg’; document.body.appendChild(myImage); |
Setting Image Attributes
Once an image object is created, developers have the ability to tailor its attributes according to their requirements. Key attributes that can be modified include the source (src), alternative text (alt), and dimensions (width and height) of the image. The following code snippet demonstrates how to set these attributes:
myImage.alt = ‘A descriptive text for the image’; myImage.width = 100; myImage.height = 100; |
Integration and Manipulation
Beyond setting basic attributes, image objects offer a plethora of possibilities for integration and manipulation within web projects. Some common operations include:
- Event Handling: Image objects can be equipped with event handlers to trigger actions based on user interactions such as clicks or mouse movements.
- Dynamic Loading: Images can be loaded dynamically, allowing developers to enhance user experience by loading images on demand rather than upfront.
- Canvas Rendering: Image objects can be rendered onto HTML canvas elements, enabling complex visual effects and transformations.
- Animation: Through JavaScript, images can be animated by altering their position, opacity, or other attributes over time.
- Filtering and Effects: Image manipulation libraries and APIs can be utilized to apply filters, effects, or transformations to images dynamically.
Best Practices
To ensure optimal performance and accessibility when working with image objects in JavaScript, consider the following best practices:
- Optimize Image Size: Resize and compress images to minimize load times and reduce bandwidth usage.
- Provide Alternative Text: Always include descriptive alternative text (alt attribute) for images to improve accessibility and facilitate understanding for users with disabilities or when images fail to load.
- Cache Images: Utilize browser caching mechanisms to store images locally, enhancing loading speed for returning visitors.
- Progressive Loading: Implement progressive loading techniques to prioritize the display of crucial content while images are being fetched.
- Responsive Design: Design web pages with responsive layouts that adapt gracefully to various screen sizes and orientations, ensuring images are displayed appropriately across devices.
JS Images: Bringing Web Pages to Life
In the expansive landscape of web development, JavaScript (JS) images stand as a powerful tool for injecting life and interactivity into web pages. From the rudimentary task of embedding static images to the sophisticated choreography of dynamic image galleries, the utilization of JS images opens up a realm of creative possibilities for developers. Let’s delve deeper into the intricacies of integrating and manipulating images within the web environment, exploring techniques, best practices, and the transformative impact of JS imagery.
Understanding JS Images
At its core, JS image manipulation involves the dynamic integration and modification of image elements within the Document Object Model (DOM) of a web page. This encompasses a diverse array of tasks, including:
- Displaying Images: Dynamically adding images to web pages by creating image objects and appending them to specific DOM elements.
- Dynamic Image Galleries: Crafting interactive galleries that enable users to navigate through a collection of images seamlessly.
- Image Sliders: Implementing sliders to showcase a sequence of images, offering a visually engaging method of presenting content.
By mastering the techniques associated with JS images, developers can imbue their web projects with visual richness and user engagement, elevating the overall browsing experience.
The Anatomy of JS Image Integration
Let’s dissect the process of integrating JS images into web pages, focusing on key components and methodologies:
- Image Object Creation: Begin by creating a new image object using the new Image() constructor in JavaScript. This serves as the foundation for subsequent image manipulation.
- Attribute Assignment: Set attributes such as the image source (src), alternative text (alt), dimensions (width and height), and other properties as needed. This allows for customization and optimization of image presentation.
- DOM Manipulation: Append the image object to a designated DOM element using methods like appendChild(), ensuring proper placement within the web page structure.
- Event Handling: Implement event listeners to facilitate user interaction with images, enabling actions such as image cycling, zooming, or navigation within galleries.
By following this systematic approach, developers can seamlessly integrate JS images into their web projects, fostering a cohesive and immersive user experience.
Best Practices for Optimal Image Management
To maximize the effectiveness and efficiency of JS image integration, adherence to best practices is essential:
- Image Optimization: Prioritize image optimization techniques such as resizing, compression, and format selection to minimize file size and improve loading times.
- Responsive Design: Design web pages with responsiveness in mind, ensuring that images adapt fluidly to various screen sizes and device orientations.
- Accessibility Enhancement: Provide descriptive alternative text (alt attributes) for images to enhance accessibility for users with disabilities and improve SEO.
- Progressive Loading: Implement progressive loading strategies to prioritize the display of essential content while images are being fetched, enhancing perceived performance.
- Cache Management: Leverage browser caching mechanisms to store images locally, reducing server load and accelerating subsequent page loads for returning visitors.
By adhering to these best practices, developers can optimize the performance, accessibility, and user experience of JS images within their web projects, ultimately driving engagement and satisfaction among site visitors.
How to Change Image Src in JavaScript
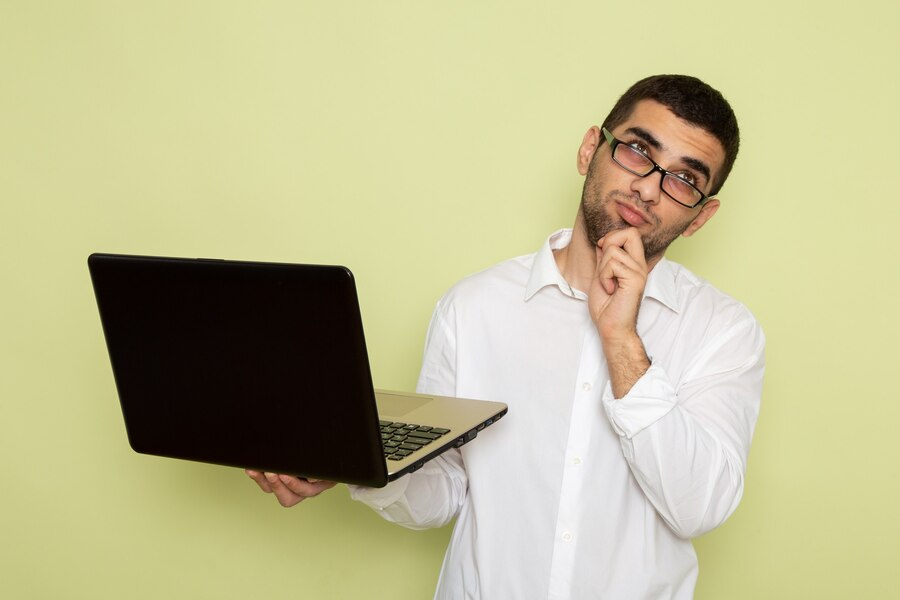
One of the most common tasks when working with images in JavaScript is changing the image’s source (src). This can be done easily by modifying the src attribute of an image object.
Understanding the Process
Changing the src attribute of an image in JavaScript involves a series of steps that are both straightforward and powerful:
- Select the Image Element: The first step is to identify and select the image element that requires modification. This is achieved using JavaScript DOM manipulation methods such as getElementById or querySelector, which enable developers to pinpoint specific elements within the DOM structure.
- Update the Source Attribute: Once the target image element is selected, the src attribute is updated by assigning it a new value. This triggers the browser to fetch and render the image content associated with the new src value, effectively replacing the existing image displayed on the web page.
A Step-by-Step Guide
Let’s break down the process into actionable steps for clarity:
- Selecting the Image Element: Use getElementById to select the image element by its unique ID. Alternatively, utilize querySelector to select the image based on CSS selectors.
- Updating the Source Attribute: Assign a new value to the src attribute of the selected image element.
document.getElementById(‘myImage’).src = ‘new/path/to/image.jpg’; |
In this example, the image with the ID ‘myImage’ has its src attribute changed to ‘new/path/to/image.jpg’, resulting in the display of a new image on the web page.
Practical Use Cases
The ability to change image src dynamically using JavaScript lends itself to a multitude of practical applications:
- Interactive Image Galleries: Implementing image galleries where users can navigate through a series of images by clicking or hovering, with each interaction triggering a change in image src.
- Profile Picture Updates: In social networking platforms or user-centric applications, allowing users to upload new profile pictures and instantly see the updated image displayed by dynamically changing the src attribute.
- Dynamic Content Loading: Loading different images based on user preferences, language settings, or contextual factors, ensuring a personalized browsing experience tailored to individual users.
Best Practices and Considerations
To optimize the effectiveness and efficiency of image src manipulation in JavaScript, developers should adhere to best practices such as:
- Error Handling: Implement robust error handling mechanisms to gracefully manage scenarios where image resources are unavailable or fail to load.
- Performance Optimization: Prioritize image optimization techniques such as compression and lazy loading to minimize page load times and enhance overall performance.
- Accessibility: Ensure that alternative text (alt attribute) is provided for images to improve accessibility and assistive technology compatibility.
Adaptive Image Loading
JavaScript empowers developers to dynamically load different images based on factors such as screen size or device resolution. This technique, known as adaptive image loading, enhances user experience by ensuring that the appropriate image is delivered to each user, optimizing bandwidth usage and improving load times, especially for mobile users. hThe steps for implementation include:
Define Multiple Image Sources
Prepare various versions of your images, each tailored for specific screen sizes and resolutions. This involves creating images in different dimensions or resolutions to accommodate various devices.
Image Type | Dimensions/Resolution |
Small Image | 300px x 200px |
Medium Image | 600px x 400px |
Large Image | 1200px x 800px |
Use JavaScript to Detect Screen Size
JavaScript provides access to the window.innerWidth property, which returns the width of the browser’s content area. By utilizing this property, you can dynamically adjust the image loading based on the detected screen size.
Dynamically Set the Image Source
Depending on the screen size detected, set the appropriate image source dynamically using JavaScript. This involves accessing the image object and updating its src attribute accordingly.
var imgElement = document.getElementById(‘responsiveImage’); if (window.innerWidth < 600) { imgElement.src = ‘path/to/small/image.jpg’; } else { imgElement.src = ‘path/to/large/image.jpg’; } |
In this example, the code retrieves an image element with the ID ‘responsiveImage’. It then checks the window’s inner width, and if it’s less than 600 pixels, it sets the source of the image element to a small image. Otherwise, it sets it to a large image.
Benefits of Adaptive Image Loading
- Bandwidth Optimization: By delivering appropriately sized images, adaptive image loading reduces unnecessary data transfer, conserving bandwidth for users.
- Improved Page Load Times: Serving optimized images enhances page load speed, leading to better user engagement and satisfaction.
- Enhanced User Experience: Users receive images tailored to their device capabilities, resulting in a seamless browsing experience across different devices and screen sizes.
Conclusion
The ability to manipulate the image object in JavaScript opens up a world of possibilities for dynamic web development. From simple tasks like how to change img src in JS to creating intricate image galleries, JavaScript provides a robust platform for creative expression in web design. By mastering these skills, developers can greatly enhance the visual appeal and interactivity of web pages, keeping users engaged and intrigued.
FAQ
A1: Yes, you can set the src attribute of an image object to an external URL.
A2: You can use the onload event of the image object. Example: myImage.onload = function() { /* Code to execute after image loads */ };
A3: Absolutely! Just set the src attribute to the base64 string: image.src = ‘data:image/jpeg;base64,…’;
A4: Yes, you can use the HTML canvas element along with JavaScript to manipulate image pixels.
A5: Use the onerror event: myImage.onerror = function() { /* Error handling code */ };
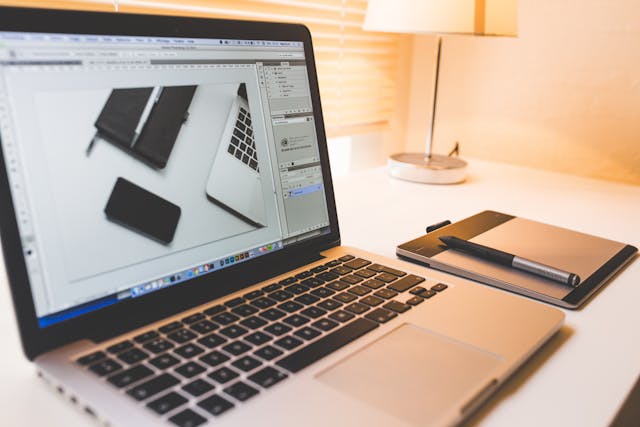
